Table of Contents
- GitHub - Wanderson-Magalhaes/Python_PySide2_Circular_ProgressBar_Modern_GUI
- GitHub - Wanderson-Magalhaes/Python_PySide2_Circular_ProgressBar_Modern_GUI
- How To Create Gui Calendar Using Python Python Tkinter Gui Project - Vrogue
- Build Your Own GUI CALENDAR with Tkinter in Python | Python Projects ...
- Introduction: Python defines an inbuilt module calendar that handles ...
- GUI Calendar using Python -2 - YouTube
- Calendar GUI using Python
- Make Calendar using GUI(Python). A calendar with a GUI using Python is ...
- GUI calendar using python and Tkinter \ Basic GUI project using python ...
- How to create GUI calendar using python - YouTube
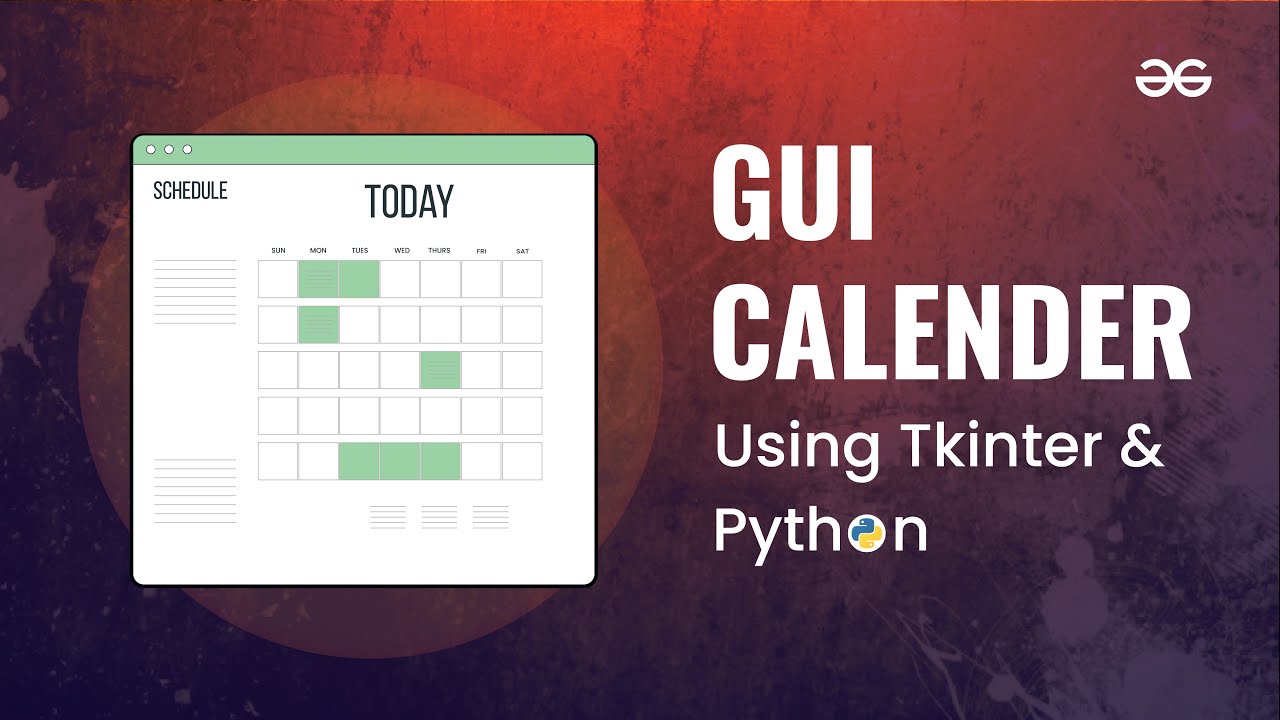

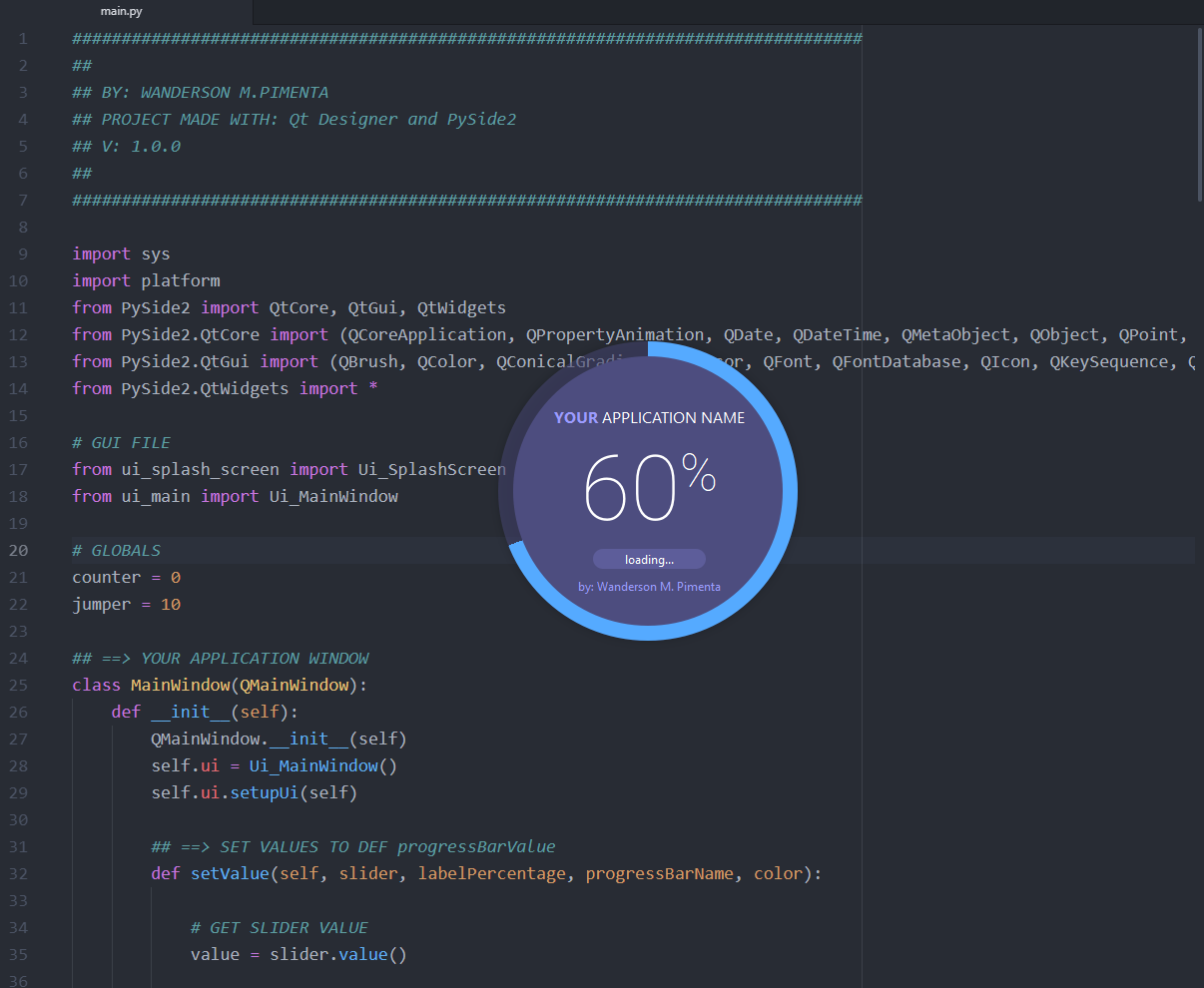
Introduction to Calendar GUI


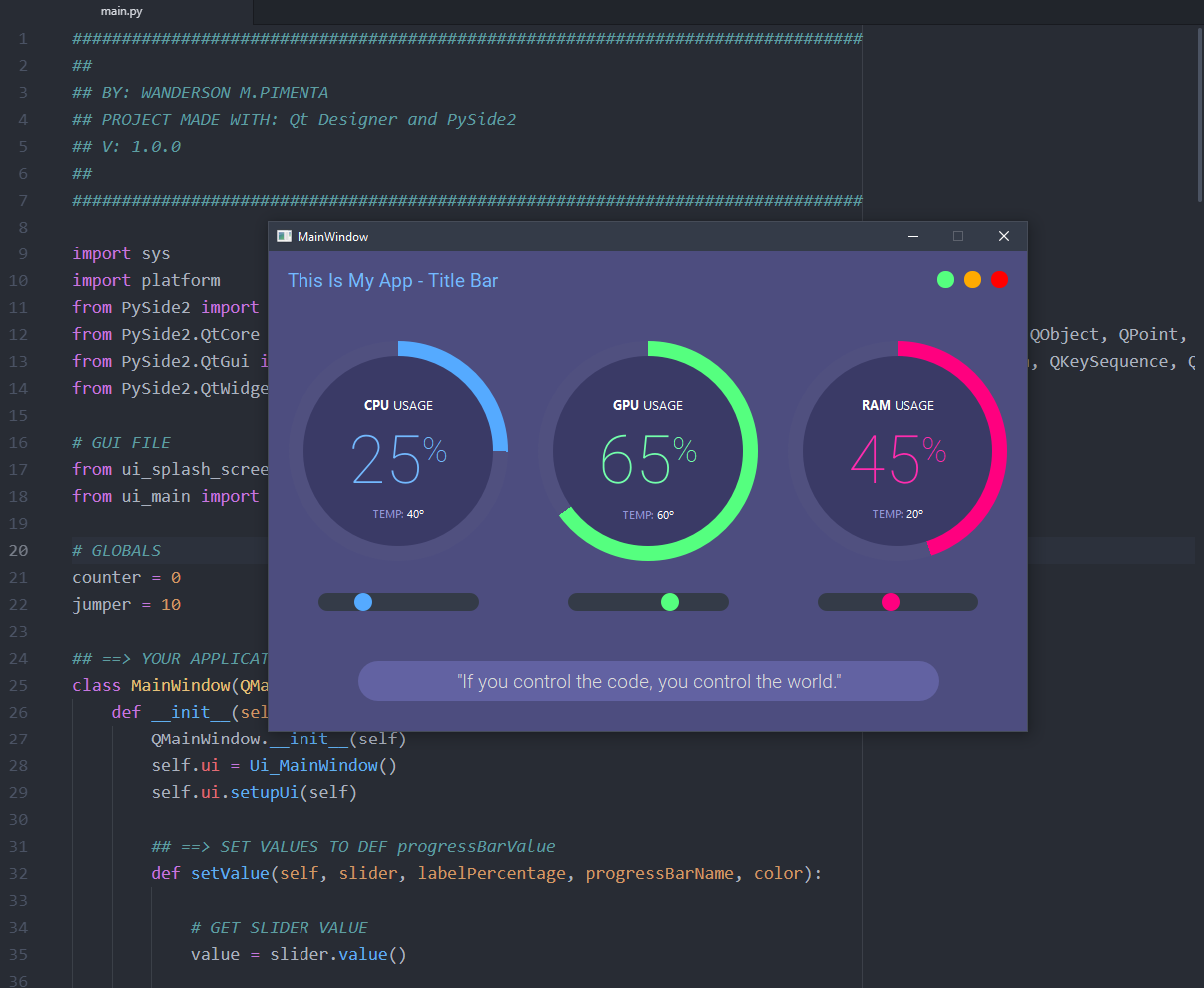
Required Libraries and Modules

tkinter
: a Python binding to the Tk GUI toolkit
calendar
: a built-in Python module for working with calendars
datetime
: a built-in Python module for working with dates and times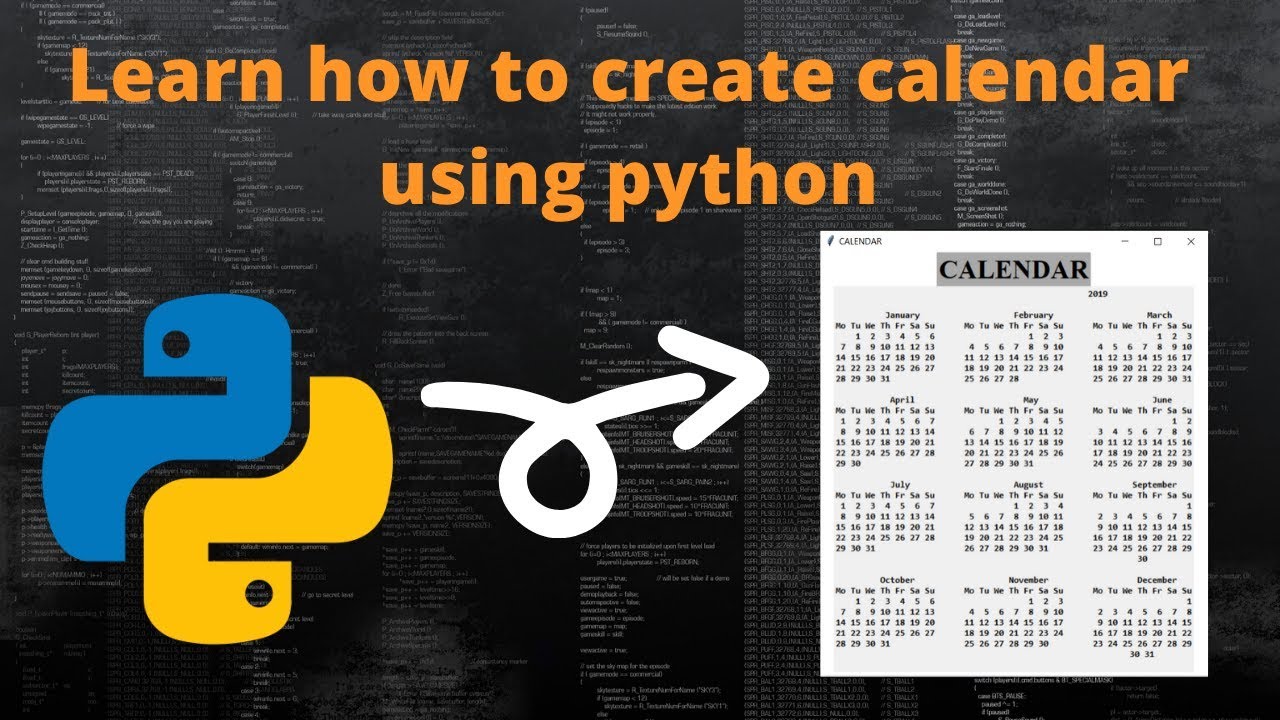
calendar
and datetime
modules are built-in, so you don't need to install them separately.
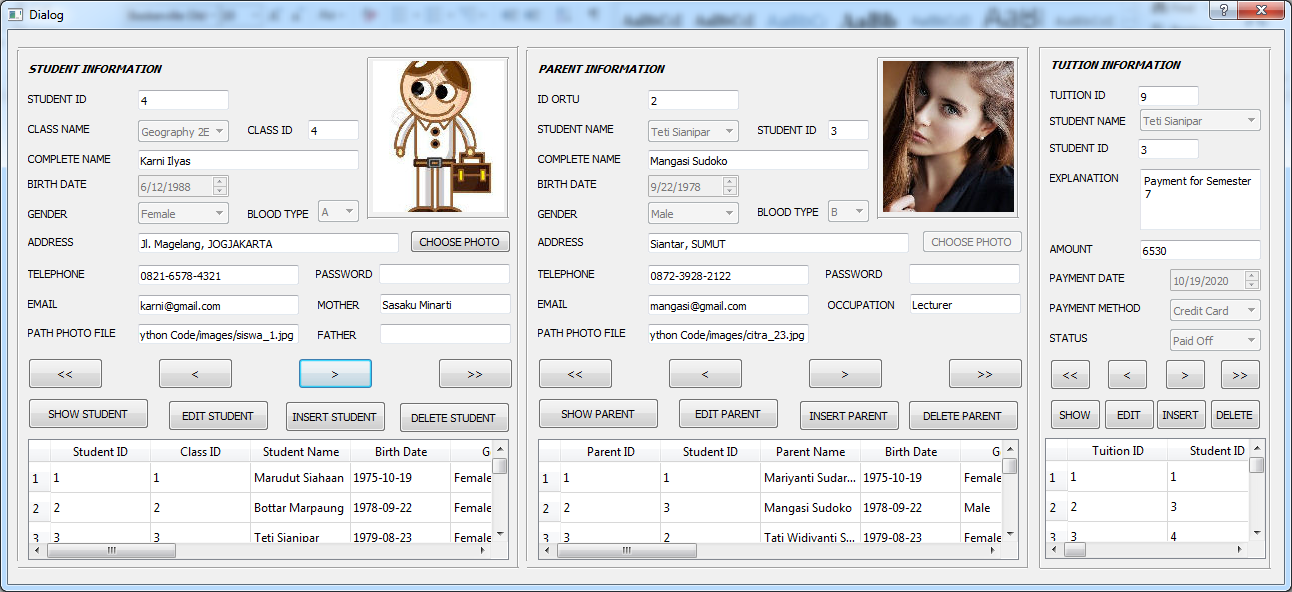
Designing the Calendar GUI
To design the calendar GUI, we will use thetkinter
library. We will create a window with a calendar layout, including buttons for navigating to different months and years.
Here is an example code snippet:
```python
import tkinter as tk
from calendar import monthrange
from datetime import date
class CalendarGUI:
def __init__(self):
self.window = tk.Tk()
self.window.title("Calendar GUI")
self.year = date.today().year
self.month = date.today().month
# Create calendar layout
self.calendar_frame = tk.Frame(self.window)
self.calendar_frame.pack()
# Create buttons for navigating to different months and years
self.button_frame = tk.Frame(self.window)
self.button_frame.pack()
self.prev_month_button = tk.Button(self.button_frame, text="Previous Month", command=self.prev_month)
self.prev_month_button.pack(side=tk.LEFT)
self.next_month_button = tk.Button(self.button_frame, text="Next Month", command=self.next_month)
self.next_month_button.pack(side=tk.LEFT)
self.prev_year_button = tk.Button(self.button_frame, text="Previous Year", command=self.prev_year)
self.prev_year_button.pack(side=tk.LEFT)
self.next_year_button = tk.Button(self.button_frame, text="Next Year", command=self.next_year)
self.next_year_button.pack(side=tk.LEFT)
# Create calendar grid
self.calendar_grid = tk.Frame(self.calendar_frame)
self.calendar_grid.pack()
# Populate calendar grid with days
self.populate_calendar()
def populate_calendar(self):
# Clear calendar grid
for widget in self.calendar_grid.winfo_children():
widget.destroy()
# Get number of days in month
num_days = monthrange(self.year, self.month)[1]
# Create day buttons
for day in range(1, num_days + 1):
day_button = tk.Button(self.calendar_grid, text=str(day), command=lambda day=day: self.day_click(day))
day_button.grid(row=(day - 1) // 7, column=(day - 1) % 7)
def day_click(self, day):
# Handle day click event
print(f"Day {day} clicked")
def prev_month(self):
# Handle previous month button click event
if self.month == 1:
self.month = 12
self.year -= 1
else:
self.month -= 1
self.populate_calendar()
def next_month(self):
# Handle next month button click event
if self.month == 12:
self.month = 1
self.year += 1
else:
self.month += 1
self.populate_calendar()
def prev_year(self):
# Handle previous year button click event
self.year -= 1
self.populate_calendar()
def next_year(self):
# Handle next year button click event
self.year += 1
self.populate_calendar()
def run(self):
self.window.mainloop()
if __name__ == "__main__":
calendar_gui = CalendarGUI()
calendar_gui.run()
```
This code creates a window with a calendar layout, including buttons for navigating to different months and years. When a day is clicked, it prints a message to the console indicating which day was clicked.
In this article, we have explored how to create a calendar GUI using Python, with the help of Aman Kharwal, also known as thecleverprogrammer. We have designed a calendar GUI with a user-friendly interface, including buttons for navigating to different months and years. We have also handled day click events and populated the calendar grid with days.
By following this tutorial, you can create your own calendar GUI using Python. You can customize the appearance and behavior of the GUI to suit your needs. With this knowledge, you can create a wide range of calendar-based applications, from simple scheduling tools to complex event management systems.
I hope this article has been helpful in guiding you through the process of creating a calendar GUI using Python. If you have any questions or need further assistance, please don't hesitate to ask. Happy coding!
Meta Description: Learn how to create a calendar GUI using Python with this step-by-step guide. Design a user-friendly interface and handle day click events with ease.
Keyword: Calendar GUI, Python, tkinter, calendar, datetime.
Note: The above article is written based on general information available on the web and may require modifications based on the specific needs and context of the project. The code snippet provided is a basic example and may need to be modified to fit the specific requirements of the project.